What are JavaScript diagramming libraries?Benefits of Using JavaScript Diagramming Libraries Ease of UseInteractivityCustomizationCross-Platform CompatibilityTop 10 JavaScript Diagramming LibrariesD3.jsGoJSJointJSDiagram.jsmxGraphVis.jsCytoscape.jsMermaidKonva.jsRaphaëlEndnote JavaScript diagramming libraries are hot tools for developers who want to turn complex information into clear, interactive diagrams. These libraries let you design, customize, and manipulate them with ease. From illustrating business…
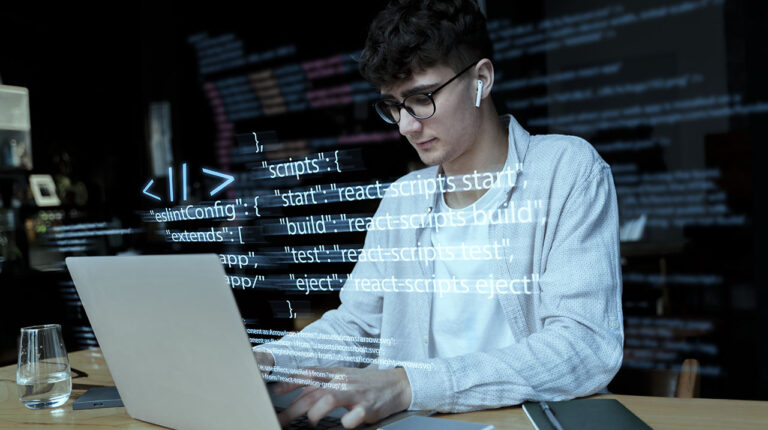