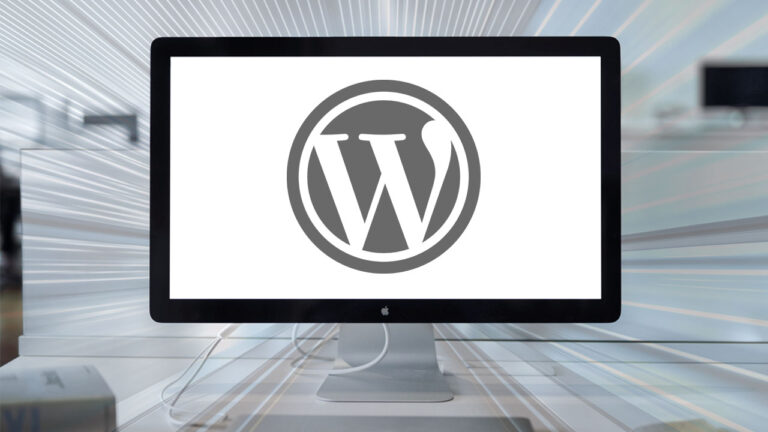
In the realm of web development, database queries play a pivotal role in determining the responsiveness and speed of your PHP applications. The art of optimizing these queries is akin to fine-tuning the engine of a high-performance vehicle – it’s essential for achieving exceptional results. In this section, we will explore a range of strategies and techniques aimed at enhancing the efficiency of your database queries within PHP applications.
Efficient database query optimization not only contributes to faster page loads and improved user experiences but also eases the burden on your server infrastructure. We’ll delve into these optimization strategies, from indexing to caching, and demonstrate how to write SQL queries that execute swiftly and with minimal resource consumption.
Let’s embark on a journey through the world of database query optimization in PHP, uncovering the key principles that will empower your applications to perform at their best.
Profiling Your PHP Code
Before diving into optimization techniques, it’s essential to understand where your application bottlenecks are. Profiling helps you identify which parts of your code are consuming the most resources. Two popular tools for profiling PHP applications are:
Xdebug Profiler
Xdebug is a powerful extension for PHP that includes a profiler. It generates detailed reports on the execution time of each function, method, and included file. To use Xdebug profiler, follow these steps:
- Install Xdebug: You can install Xdebug using
pecl
or by downloading the source code. - Configure PHP: Add the following lines to your
php.ini
file:
zend_extension=xdebug.so
xdebug.profiler_enable=1
xdebug.profiler_output_dir=/path/to/profiles
- Replace
/path/to/profiles
with your desired output directory. - Generate Profiling Data: Trigger the profiler by adding
?XDEBUG_PROFILE=1
to your application’s URL. - Analyze the Data: Analyze the generated profile files using tools like KCacheGrind, WebGrind, or Xdebug Profiler Viewer.
Blackfire
Blackfire is a premium performance profiling tool that offers deep insights into your PHP applications. It provides a user-friendly interface and integration with popular PHP frameworks. To get started with Blackfire:
- Sign Up: Create an account on the Blackfire website.
- Install the Blackfire Agent: Follow the installation instructions for your specific platform.
- Configure PHP: Add the following lines to your
php.ini
:
extension=blackfire.so
blackfire.agent_socket=tcp://127.0.0.1:8707
blackfire.server_id=YOUR_SERVER_ID
blackfire.server_token=YOUR_SERVER_TOKEN
- Replace
YOUR_SERVER_ID
andYOUR_SERVER_TOKEN
with your Blackfire credentials. - Profile Your Code: Use the Blackfire web interface or command-line tool to profile your PHP applications.
Optimize Database Queries
Database queries often constitute a significant portion of a web application’s execution time. Optimizing your database queries can lead to substantial performance improvements. Let’s explore the various strategies for optimizing database queries in PHP:
1. Indexing
One of the most effective ways to optimize database queries is by using indexes. Indexes are data structures that provide a quick way to look up data based on the values in one or more columns. Here’s how to use indexing effectively:
- Identify Query Bottlenecks: Use profiling tools or the database’s built-in tools to identify slow-running queries. Pay attention to queries that involve large tables or lack proper indexing.
- Add Indexes: Analyze the queries and add indexes to the columns used in the
WHERE
,JOIN
, andORDER BY
clauses. However, avoid over-indexing, as too many indexes can slow down INSERT and UPDATE operations. - Composite Indexes: Consider using composite indexes (indexes on multiple columns) when queries involve multiple conditions. A well-designed composite index can significantly improve query performance.
- Regularly Maintain Indexes: Over time, as data changes, indexes may become fragmented. Regularly maintain indexes by rebuilding or reorganizing them to ensure optimal performance.
2. Caching
Caching query results can reduce the load on the database and improve query response times. There are two primary types of caching:
- Query Result Caching: Store the results of frequently executed queries in memory or a caching system like Memcached or Redis. When the same query is requested again, retrieve the results from the cache instead of executing the query.
- Object Caching: For complex queries that involve multiple tables or require data processing, consider caching entire objects or data structures rather than just query results. This can be especially beneficial for read-heavy applications.
3. Using Prepared Statements
Prepared statements are a security feature, but they can also improve query performance. Here’s how:
- Reduced Query Parsing Overhead: Prepared statements are precompiled by the database server, reducing the parsing overhead for each query execution. This can lead to faster query execution, especially for queries that are executed multiple times with different parameters.
- Protection Against SQL Injection: Prepared statements automatically escape and sanitize input data, providing an additional layer of security by preventing SQL injection attacks.
- Parameter Binding: Prepared statements allow you to bind parameters to query placeholders, which can optimize query execution plans by allowing the database to reuse query plans for similar queries with different parameter values.
4. Query Optimization
Query optimization involves writing efficient SQL queries that retrieve the required data with minimal overhead. Consider the following tips:
- Select Only What You Need: Avoid using
SELECT *
when querying the database. Instead, specify the specific columns you need. Retrieving unnecessary data can lead to increased I/O and slower query execution. - Limit and Offset: When dealing with large result sets, use
LIMIT
andOFFSET
clauses to retrieve only a subset of records at a time. This can improve query response times and reduce memory consumption. - Avoid Subqueries When Possible: Subqueries can be resource-intensive. Whenever possible, rewrite queries to use joins or other techniques that can achieve the same results more efficiently.
By implementing these database query optimization strategies, you can significantly improve the performance of your PHP applications, especially when dealing with large datasets or high query loads. Remember that profiling and benchmarking are essential for measuring the impact of optimizations accurately.
Code-Level Optimizations To Boost PHP Applications
Optimizing your PHP code at the source level is essential for achieving significant performance gains. Let’s explore several key strategies in detail:
1. Minimize Database Queries
Database interactions often constitute a significant portion of a web application’s execution time. To optimize them:
- Combine Multiple Queries: Instead of making several separate database queries, consolidate them into a single query. This reduces the overhead of connecting to the database and executing queries multiple times.
- Efficient JOINs: When using JOIN operations to fetch related data from multiple tables, ensure that you use appropriate indexes on the join columns. This speeds up the data retrieval process.
- SELECT Only What You Need: Avoid using
SELECT *
when querying the database. Retrieve only the specific columns you need, reducing the amount of data transferred between the database and the application.
2. Optimize Loops
Loops are fundamental in programming, but they can be resource-intensive, especially when processing large datasets. Here’s how to optimize them:
- Favor
foreach
overfor
: When iterating over arrays or collections,foreach
loops are often more efficient and easier to read than traditionalfor
loops. PHP’s internal optimizations makeforeach
loops faster for array traversal. - Early Loop Termination: In some cases, you can exit a loop early if a certain condition is met. This can save processing time by avoiding unnecessary iterations.
3. Efficient Data Structures
Choosing the right data structure can significantly impact performance:
- Indexed Arrays: When working with simple lists of data, indexed arrays are generally more memory-efficient and faster to access than associative arrays or objects.
- Associative Arrays for Quick Lookups: If you need to perform quick lookups based on keys, associative arrays or hash tables are suitable. However, keep in mind that they may consume more memory than indexed arrays.
4. Avoid Costly Functions
Some PHP functions are inherently more resource-intensive than others. To optimize your code, avoid using functions with high overhead when simpler alternatives are available:
- Avoid
eval()
: Theeval()
function is powerful but can be a significant performance bottleneck. It interprets and executes PHP code dynamically, which is slow. Whenever possible, find alternatives to dynamic code execution. - Minimize Regular Expressions: Regular expressions (regex) can be CPU-intensive. If you’re using regex extensively, evaluate whether you can optimize or simplify your patterns to improve performance.
These code-level optimizations can have a substantial impact on your PHP application’s performance. Remember to profile your code before and after making changes to measure the improvements accurately.
Caching Strategies
Caching is a powerful technique to reduce server load and enhance the responsiveness of your PHP application. Here’s a detailed look at caching strategies:
1. Opcode Caching
Opcode caches like APCu, OPcache, or XCache store precompiled script bytecode in memory. This prevents PHP from recompiling the same scripts on each request, leading to faster execution times. To implement opcode caching:
- Enable and Configure OPcache: Most modern PHP distributions come with OPcache preinstalled. Ensure it’s enabled in your PHP configuration (
php.ini
). You can configure it with settings likeopcache.memory_consumption
andopcache.validate_timestamps
.
2. Content Caching
Content caching involves storing the HTML output of web pages and serving it to subsequent users without regenerating the content. Key points include:
- Use a Cache Store: Popular cache stores like Memcached and Redis are excellent choices for content caching. They allow you to store and retrieve cached data efficiently.
- Implement Cache Expiration: Define a strategy for when cached content should expire. This ensures that users receive up-to-date information.
3. Page Caching
Page caching takes caching a step further by saving entire web pages as static files and serving them directly to users. To implement page caching:
- Use a Reverse Proxy: Tools like Varnish or NGINX can act as reverse proxies and cache entire pages. They can dramatically reduce server load and response times by serving cached pages without invoking PHP.
By intelligently applying these caching strategies, you can significantly reduce the time it takes to generate and serve content, resulting in faster and more responsive web applications.
Server-Side Enhancements
Optimizing your server-side environment can have a substantial impact on PHP application performance. Here’s a closer look at server-side enhancements:
1. Use a Content Delivery Network (CDN)
A Content Delivery Network (CDN) is a distributed network of servers that store cached copies of your website’s static assets, such as images, stylesheets, and JavaScript files. Leveraging a CDN offers several benefits:
- Reduced Latency: CDNs have servers in various geographic locations, enabling them to serve content from a server that is physically closer to the user. This minimizes the time it takes for data to travel and results in faster page loading times.
- Load Balancing: CDNs often provide load balancing, distributing user requests across multiple servers to ensure optimal performance and redundancy.
2. Enable GZIP Compression
GZIP compression reduces the size of data transferred between the server and the client. When enabled, the server compresses response data before sending it to the client, which then decompresses it for rendering. Benefits of enabling GZIP compression include:
- Faster Page Loading: Smaller data payloads lead to faster page loading times, particularly for users on slower network connections.
- Reduced Bandwidth Usage: Compressed data consumes less bandwidth, which can be cost-effective, especially when hosting high-traffic websites.
3. Load Balancing
Load balancing involves distributing incoming web traffic across multiple server instances. This helps prevent overloading a single server and provides redundancy in case one server fails. Key considerations include:
- Scalability: Load balancing facilitates easy scaling of your infrastructure to accommodate increased traffic as your application grows.
- Fault Tolerance: In case one server experiences issues or downtime, load balancing ensures that users are directed to healthy servers, minimizing service interruptions.
These server-side enhancements, combined with code-level optimizations and caching strategies, contribute to a well-rounded approach for optimizing PHP application performance.
Conclusion
Optimizing PHP performance is a continuous process that requires careful planning and regular monitoring. By applying code-level optimizations, implementing effective caching strategies, and enhancing your server-side environment, you can significantly improve the speed and responsiveness of your PHP applications. Remember to balance performance improvements with code maintainability and test thoroughly to ensure that optimizations do not introduce new issues. Stay tuned for more in-depth guides and tools to help fine-tune your PHP applications for optimal performance.