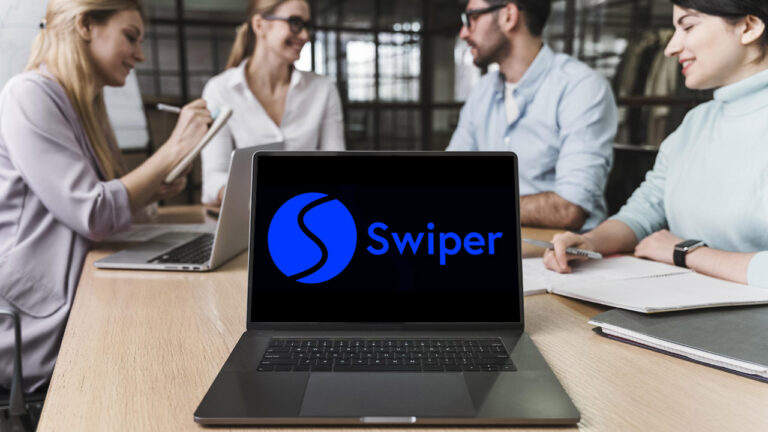
Table of Contents
Introduction to Swiper.js
What is Swiper.js?
Swiper.js is a powerful and versatile JavaScript library that enables developers to create modern, touch-friendly sliders and carousels. It is designed to work seamlessly on both desktop and mobile devices, providing a smooth and responsive experience. Swiper.js is widely used in web development due to its rich feature set, ease of integration, and extensive customization options. Whether you’re building a simple image carousel, a complex multi-slide layout, or an interactive full-page slider, Swiper.js offers the tools you need to create visually appealing and highly functional sliders. For those looking to get started with learning and implementing web development technologies, Tutor Map can be a valuable resource for finding relevant tutors and resources.
Key Features and Benefits
Swiper.js stands out for its comprehensive features and numerous benefits that make it a popular choice among web developers. Here are some of the key features and benefits:
Touch Control
Swiper.js is optimized for touch interactions, making it an ideal choice for mobile-first and responsive web designs. It supports touch gestures such as swiping, which allows users to navigate through slides effortlessly. This feature is particularly valuable for mobile users who rely on touch interactions for navigation. Additionally, Swiper.js supports mouse interactions, ensuring a consistent experience across all devices.
Responsive Design
Swiper.js automatically adjusts to different screen sizes, ensuring that sliders look great on all devices, from large desktop monitors to small mobile screens. The library provides several responsive design options, allowing developers to specify different settings based on the screen size. This flexibility ensures that the slider’s appearance and functionality remain consistent and optimized for any device, enhancing the overall user experience.
Customizability
One of the standout features of Swiper.js is its high degree of customizability. Developers can configure various aspects of the slider, including its behavior, appearance, and layout. Swiper.js offers a wide range of configuration options, such as autoplay, loop mode, speed, direction, and more. Additionally, it provides numerous callbacks and events that allow developers to execute custom code at different stages of the slider’s lifecycle. This level of customization makes it easy to tailor Swiper.js to fit the specific needs of any project.
Performance
Swiper.js is designed with performance in mind. It is lightweight and efficient, ensuring fast loading times and smooth animations. The library’s optimized code minimizes the impact on page load speed, which is crucial for maintaining a positive user experience. Swiper.js also supports features like lazy loading, which delays the loading of images until they are needed, further enhancing performance and reducing initial load times. These performance optimizations make Swiper.js an excellent choice for creating high-performing sliders and carousels.
By offering these key features and benefits, Swiper.js has become a go-to solution for developers looking to create engaging, responsive, and customizable sliders for their web projects. In the following sections, we will explore how to set up and use Swiper.js, customize its behavior, and implement advanced features to create stunning sliders that enhance the user experience.
Setting Up Swiper.js
Prerequisites
Before diving into Swiper.js, it is essential to have a basic understanding of HTML, CSS, and JavaScript. These skills will help you effectively integrate and customize Swiper.js in your web projects.
- HTML: Knowledge of HTML is necessary to create the structure of your sliders.
- CSS: Familiarity with CSS will allow you to style your sliders and ensure they look visually appealing.
- JavaScript: Understanding JavaScript is crucial for initializing Swiper.js and configuring its behavior.
Installation Options
Swiper.js offers multiple installation methods to suit different development environments and preferences. Here are three common ways to install Swiper.js:
Using a CDN
One of the simplest ways to include Swiper.js in your project is by using a Content Delivery Network (CDN). This method requires no additional setup and allows you to quickly start using Swiper.js by linking to the CDN-hosted files.
- Include the CSS file: Add the following link tag in the
<head>
section of your HTML document.<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
- Include the JS file: Add the following script tag before the closing
</body>
tag of your HTML document.<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
Installing via npm/yarn
For projects using a build system or module bundler like Webpack or Parcel, you can install Swiper.js via npm or yarn. This method provides better control over dependencies and version management.
- Install Swiper.js using npm:
npm install swiper
- Or install Swiper.js using yarn:
yarn add swiper
- Import Swiper.js in your JavaScript file:
import Swiper from 'swiper/bundle';
import 'swiper/swiper-bundle.css';
Downloading and Hosting Locally
You can also download Swiper.js files and host them locally in your project. This method is useful if you prefer not to rely on external resources or need offline access.
- Download the Swiper.js package: Go to the Swiper.js GitHub repository and download the latest release.
- Extract the files: Unzip the downloaded package and locate the CSS and JS files.
- Include the CSS file: Add the following link tag in the
<head>
section of your HTML document, pointing to the locally hosted CSS file.<link rel="stylesheet" href="path/to/swiper-bundle.min.css">
- Include the JS file: Add the following script tag before the closing
</body>
tag of your HTML document, pointing to the locally hosted JS file.<script src="path/to/swiper-bundle.min.js"></script>
Adding Swiper.js to Your Project
Once you have chosen and implemented one of the installation methods, you can add Swiper.js to your project by including the necessary CSS and JS files.
- Include the CSS file:
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
- Include the JS file:
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
Or, if you are using a module bundler:
- Import the CSS file in your JavaScript file:
import 'swiper/swiper-bundle.css';
- Import Swiper.js in your JavaScript file:
import Swiper from 'swiper/bundle';
Basic Swiper.js Setup
Creating the Basic HTML Structure
To get started with Swiper.js, you need to create the basic HTML structure that will hold your slider. This involves creating a container for the Swiper instance and adding individual slides within this container.
HTML Markup for the Swiper Container and Slides
- Swiper Container: The outer container that will hold all the slides.
- Swiper Wrapper: A div inside the container that wraps around all the slides.
- Swiper Slides: Individual slide elements inside the wrapper.
Here’s the basic HTML structure for a Swiper slider:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Swiper.js Basic Setup</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<!-- Swiper Container -->
<div class="swiper-container">
<!-- Additional required wrapper -->
<div class="swiper-wrapper">
<!-- Slides -->
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
<!-- ... -->
</div>
<!-- If you want pagination (bullets) -->
<div class="swiper-pagination"></div>
<!-- If you want navigation buttons -->
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
<!-- If you want a scrollbar -->
<div class="swiper-scrollbar"></div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
// Your JavaScript will go here
</script>
</body>
</html>
In this structure:
- The
.swiper-container
class is the main container for the Swiper slider. - The
.swiper-wrapper
class wraps around all the slide elements. - Each slide has the
.swiper-slide
class.
Initializing Swiper.js
Once you have the basic HTML structure in place, the next step is to initialize Swiper.js with JavaScript. This involves creating a new Swiper instance and passing it the container selector and any desired configuration options.
Basic JavaScript to Initialize a Swiper Instance
Here’s how to initialize Swiper.js using JavaScript:
- Create a new Swiper instance: Use the Swiper constructor and pass it the CSS selector for the container and an optional configuration object.
- Example Initialization:
<script>
document.addEventListener('DOMContentLoaded', function () {
var swiper = new Swiper('.swiper-container', {
// Optional parameters
direction: 'horizontal', // 'horizontal' or 'vertical'
loop: true, // Loop through slides
// If you want pagination (bullets)
pagination: {
el: '.swiper-pagination',
clickable: true,
},
// If you want navigation buttons
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
// If you want a scrollbar
scrollbar: {
el: '.swiper-scrollbar',
},
});
});
</script>
This script initializes a basic Swiper instance with the following options:
- Direction: Set to
horizontal
, making the slides move horizontally. You can change it tovertical
if you prefer vertical sliding. - Loop: Set to
true
, allowing the slides to loop infinitely. - Pagination: Adds clickable pagination bullets.
- Navigation: Adds next and previous navigation buttons.
- Scrollbar: Adds a scrollbar for additional navigation.
Example Code Snippet
Here is a complete example combining the HTML structure and JavaScript initialization:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Swiper.js Basic Setup</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<!-- Swiper Container -->
<div class="swiper-container">
<!-- Additional required wrapper -->
<div class="swiper-wrapper">
<!-- Slides -->
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
<!-- ... -->
</div>
<!-- If you want pagination (bullets) -->
<div class="swiper-pagination"></div>
<!-- If you want navigation buttons -->
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
<!-- If you want a scrollbar -->
<div class="swiper-scrollbar"></div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
document.addEventListener('DOMContentLoaded', function () {
var swiper = new Swiper('.swiper-container', {
direction: 'horizontal',
loop: true,
pagination: {
el: '.swiper-pagination',
clickable: true,
},
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
scrollbar: {
el: '.swiper-scrollbar',
},
});
});
</script>
</body>
</html>
This example provides a basic setup for Swiper.js, including the necessary HTML structure and JavaScript initialization. You can now customize and extend your Swiper instance with various configuration options to create the desired slider experience for your project.
Configuring Swiper.js
Overview of Configuration Options
Swiper.js offers a wide range of configuration options that allow you to customize the behavior and appearance of your sliders. These options can be set when initializing the Swiper instance and provide control over aspects like looping, autoplay, speed, direction, and more. Here are some of the key configuration options:
direction
: Defines the direction of slide movement (horizontal
orvertical
).loop
: Enables continuous loop mode (true
orfalse
).autoplay
: Enables automatic sliding with a specified delay.speed
: Sets the transition speed between slides (in milliseconds).pagination
: Configures pagination (bullet navigation).navigation
: Configures navigation buttons (next and previous).scrollbar
: Configures the scrollbar for additional navigation.effect
: Changes the transition effect between slides (e.g.,slide
,fade
,cube
,coverflow
,flip
).
These options allow you to create highly customized and interactive sliders tailored to your specific needs.
Customizing the Swiper Instance
To customize the Swiper instance, you can pass an options object to the Swiper constructor. This object can include any of the available configuration options.
Examples of Different Configurations
Here are a few examples demonstrating different configurations of Swiper.js:
- Basic Horizontal Slider with Looping
var swiper = new Swiper('.swiper-container', {
direction: 'horizontal',
loop: true,
});
- Vertical Slider with Autoplay
var swiper = new Swiper('.swiper-container', {
direction: 'vertical',
autoplay: {
delay: 3000,
},
});
- Slider with Custom Speed and Navigation Buttons
var swiper = new Swiper('.swiper-container', {
speed: 800,
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
});
- Slider with Pagination and Scrollbar
var swiper = new Swiper('.swiper-container', {
pagination: {
el: '.swiper-pagination',
clickable: true,
},
scrollbar: {
el: '.swiper-scrollbar',
hide: true,
},
});
- Slider with Fade Effect
var swiper = new Swiper('.swiper-container', {
effect: 'fade',
fadeEffect: {
crossFade: true,
},
});
Example Code Snippets for Various Configurations
Here are complete examples combining HTML structure and JavaScript initialization for different configurations:
- Basic Horizontal Slider with Looping
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Basic Horizontal Slider</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper('.swiper-container', {
direction: 'horizontal',
loop: true,
});
</script>
</body>
</html>
- Vertical Slider with Autoplay
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Vertical Slider with Autoplay</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper('.swiper-container', {
direction: 'vertical',
autoplay: {
delay: 3000,
},
});
</script>
</body>
</html>
- Slider with Custom Speed and Navigation Buttons
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Slider with Navigation Buttons</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
<div class="swiper-button-prev"></div>
<div class="swiper-button-next"></div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper('.swiper-container', {
speed: 800,
navigation: {
nextEl: '.swiper-button-next',
prevEl: '.swiper-button-prev',
},
});
</script>
</body>
</html>
- Slider with Pagination and Scrollbar
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Slider with Pagination and Scrollbar</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
<div class="swiper-pagination"></div>
<div class="swiper-scrollbar"></div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper('.swiper-container', {
pagination: {
el: '.swiper-pagination',
clickable: true,
},
scrollbar: {
el: '.swiper-scrollbar',
hide: true,
},
});
</script>
</body>
</html>
- Slider with Fade Effect
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Slider with Fade Effect</title>
<link rel="stylesheet" href="https://unpkg.com/swiper/swiper-bundle.min.css">
</head>
<body>
<div class="swiper-container">
<div class="swiper-wrapper">
<div class="swiper-slide">Slide 1</div>
<div class="swiper-slide">Slide 2</div>
<div class="swiper-slide">Slide 3</div>
</div>
</div>
<script src="https://unpkg.com/swiper/swiper-bundle.min.js"></script>
<script>
var swiper = new Swiper('.swiper-container', {
effect: 'fade',
fadeEffect: {
crossFade: true,
},
});
</script>
</body>
</html>
These examples demonstrate how to configure Swiper.js to achieve various effects and functionalities. By adjusting the configuration options, you can create a wide range of slider experiences to meet your project’s needs.
Common Issues and Troubleshooting
Common Problems Beginners Might Face
When getting started with Swiper.js, beginners might encounter a variety of common issues. Here are some typical problems and how to address them:
- Initialization Issues:
- Problem: Swiper.js is not initializing properly.
- Solution: Ensure that the Swiper.js script and stylesheets are correctly included in your HTML file. Verify that the Swiper container and wrapper elements have the correct class names.
- Styling Problems:
- Problem: The slider is not displaying correctly, or slides are not visible.
- Solution: Check that the CSS is correctly applied to the Swiper container and slides. Make sure the container has a defined width and height. Verify that there are no conflicting CSS rules affecting the slider.
- JavaScript Errors:
- Problem: JavaScript errors are preventing Swiper.js from working.
- Solution: Open the browser console to check for any error messages. These messages can provide clues about what is going wrong. Ensure that there are no syntax errors in your Swiper initialization code.
- Pagination/Navigation Not Working:
- Problem: Pagination bullets or navigation buttons are not functioning.
- Solution: Verify that the pagination and navigation elements are correctly referenced in the Swiper configuration. Ensure that the elements exist in the HTML and have the correct class names.
- Autoplay Issues:
- Problem: Autoplay is not working as expected.
- Solution: Check the autoplay configuration in the Swiper initialization code. Make sure that the
autoplay
option is correctly set, and there are no conflicting settings.
Tips for Debugging
When troubleshooting issues with Swiper.js, the following tips can help you identify and resolve problems:
- Use Console Logs:
- Add
console.log
statements to your JavaScript code to track the execution flow and values of variables. This can help you pinpoint where things might be going wrong.
- Add
- Check Browser Console for Errors:
- The browser console provides detailed error messages and warnings that can help you understand what is causing an issue. Open the console (usually by pressing
F12
orCtrl+Shift+I
) and look for any errors related to Swiper.js or your custom code.
- The browser console provides detailed error messages and warnings that can help you understand what is causing an issue. Open the console (usually by pressing
- Review Swiper.js Documentation:
- The Swiper.js documentation is a comprehensive resource that covers all aspects of Swiper.js. It includes detailed explanations of configuration options, methods, and events. Refer to the documentation to ensure you are using the correct syntax and options.
- Search Online Forums and Communities:
- Online forums, such as Stack Overflow, and communities like the Swiper.js GitHub repository, can be valuable resources for troubleshooting. Search for similar issues that other developers have encountered and see how they resolved them. You can also ask questions and seek advice from the community.
- Simplify Your Code:
- If you are encountering issues, try simplifying your code to the most basic example that still reproduces the problem. This can help isolate the issue and make it easier to identify what is causing it.
- Check for Conflicting Scripts or Styles:
- Other scripts or CSS styles on your page might conflict with Swiper.js. Disable other scripts and styles temporarily to see if the issue persists. If disabling them resolves the issue, you can then narrow down which one is causing the conflict.
By following these tips and utilizing the available resources, you can effectively troubleshoot and resolve common issues when working with Swiper.js.